In the ever-evolving landscape of web development, ensuring the security of user data and controlling access to different parts of your application is paramount. Authentication and authorization are two critical components that help you achieve these goals. In this comprehensive guide, we’ll delve into the world of authentication and authorization in React applications, exploring best practices, common techniques, and tools to keep your application secure.
Getting Started with React.js
React.js, an open-source JavaScript library, is specifically designed for constructing user interfaces. Originally created by Facebook in 2011, it was subsequently released as an open-source project. React.js streamlines the creation of intricate and interactive user interfaces by decomposing them into smaller, reusable components.
Key Features of React.js
Component-Based Architecture:
At its core, React revolves around the concept of components. Each component is an independent, reusable block of code that encapsulates a specific portion of the user interface. By composing these components together, intricate user interfaces can be constructed. This component-based methodology fosters both code reusability and maintainability.Virtual DOM:
React uses a virtual representation of the DOM (Document Object Model) to optimize rendering. Instead of making direct changes to the actual DOM, React compares the virtual DOM with the real DOM and only updates the parts that have changed. This minimizes browser reflows and improves performance.Declarative Syntax:
React uses a declarative approach to define how the UI should look based on the application’s state. Developers describe the desired UI, and React takes care of updating it when the state changes. This makes code easier to understand and maintain.Unidirectional Data Flow:
React enforces a unidirectional data flow, which means that data flows in one direction from parent components to child components. This simplifies data management and helps prevent unexpected side effects.JSX:
React introduces JSX (JavaScript XML), which allows developers to write HTML-like code within JavaScript. JSX makes it easier to create and visualize the UI structure directly in the code.Rich Ecosystem:
React has a vibrant ecosystem with a wide range of libraries and tools for routing, state management, testing, and more. Popular libraries like Redux, React Router, and Material-UI complement React and extend its capabilities.Community Support:
React has a large and active community of developers. This means there are ample resources, tutorials, and third-party components available to help developers build applications efficiently.
Use Cases of React.js
React.js is applied extensively in the development of various types of applications, including
Single Page Applications (SPAs):
React is well-suited for building SPAs where content is dynamically loaded without full page refreshes. This results in a smoother and more responsive user experience.Web and Mobile Apps:
React can be used to build both web and mobile applications. With tools like React Native, developers can create native mobile apps for iOS and Android using the same codebase.User Interfaces:
React is used to create user interfaces for a wide range of applications, from e-commerce websites to social media platforms.Interactive Dashboards:
React’s component-based architecture is ideal for building interactive dashboards and data visualization tools.Content Management Systems (CMS):
React can be integrated into content management systems to provide a modern and dynamic user interface for content creators.E-commerce Platforms:
Many e-commerce websites leverage React to provide a seamless shopping experience with real-time updates and smooth navigation.
In conclusion, React.js has revolutionized the way developers build user interfaces on the web. Its component-based architecture, virtual DOM, and declarative syntax have made it a powerful and efficient tool for building dynamic and responsive web applications. With a strong community and a rich ecosystem, React continues to be a top choice for front-end development.
Also Read: Top React.js development companies in USA
Understanding Authentication and Authorization
Before we dive into the technical aspects, let’s clarify the difference between authentication and authorization
Authentication
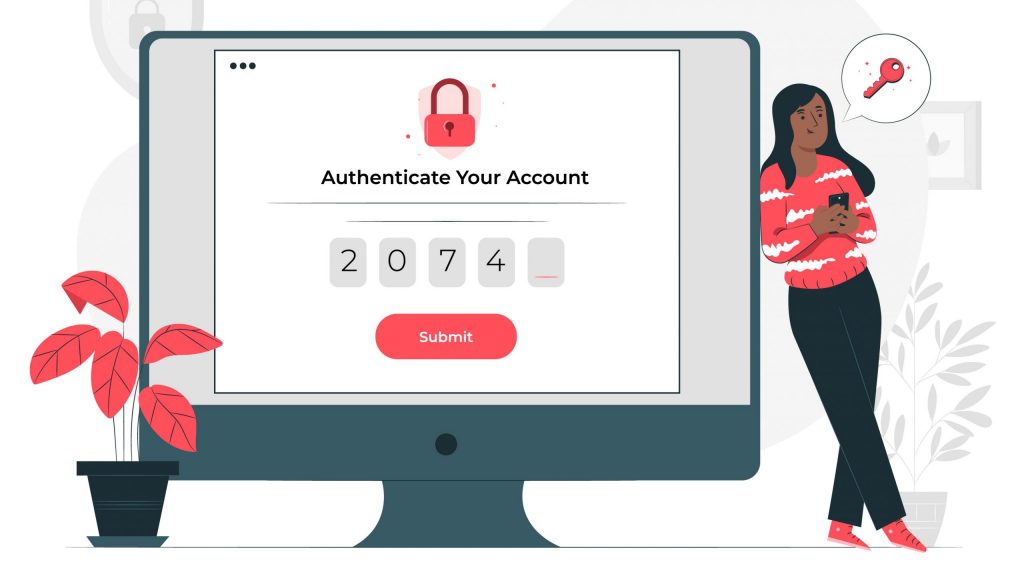
Authentication is the process of verifying the identity of a user. It answers the question, “Who are you?” Users typically provide credentials such as usernames and passwords, or use social login options like Google or Facebook to prove their identity.
User Registration and Login
Implementing authentication in a React application starts with building a user registration and login system. You can choose to build these components from scratch or leverage authentication services like Firebase or Auth0 to streamline the process.
Securely Storing User Credentials
To ensure the security of user data, it’s crucial to securely hash and store user credentials such as passwords. Never store plain text passwords in your database. Libraries like Bcrypt can help with secure password hashing.
Token-Based Authentication
Token-based authentication is a widely adopted approach. When a user logs in, the server generates a token (usually a JSON Web Token or JWT) and sends it back to the client. This token is then included in subsequent API requests to authenticate the user.
Persistent Authentication
To provide a seamless user experience, implement mechanisms for keeping users authenticated even after they close the browser or revisit the app. Technologies like cookies and localStorage can be useful for this purpose.
Authorization
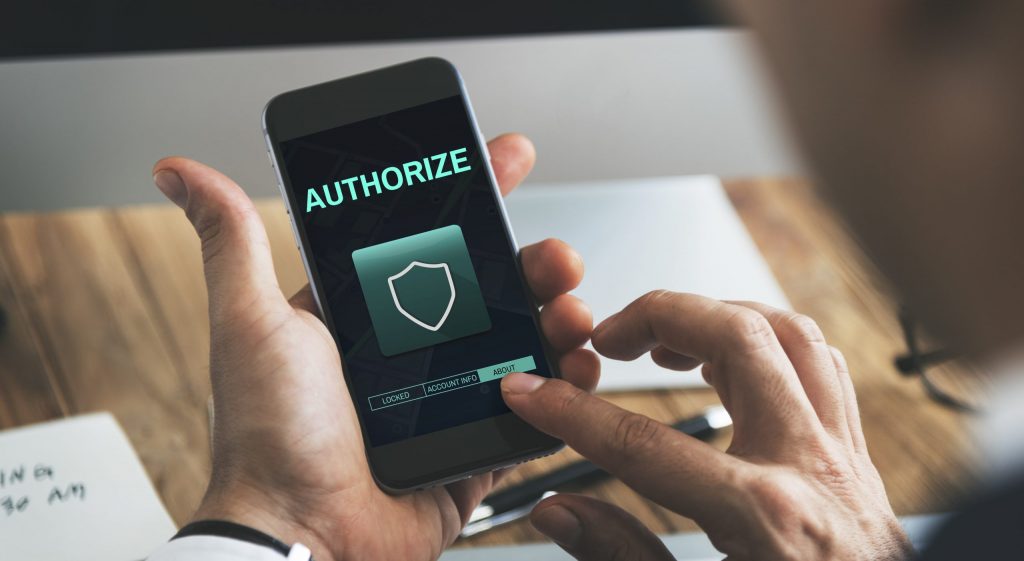
Authorization, on the other hand, is the process of determining what a user is allowed to do within an application once they are authenticated. It answers the question, “What are you allowed to do?” Authorization mechanisms define which parts of your application a user can access and what actions they can perform.
Role-Based Access Control (RBAC)
Role-Based Access Control (RBAC) is a common authorization model where users are assigned roles, and each role has specific permissions. Define roles like “admin,” “user,” or “guest” and restrict access to different parts of your app accordingly.
Conditional Rendering
In React, you can conditionally render components based on a user’s authorization level. For example, show an “Admin Panel” link only to users with admin privileges.

Middleware and Route Guards
Use middleware or route guards to protect certain routes from unauthorized access. Libraries like React Router provide a way to implement route guards easily.

Tools and Libraries for Authentication and Authorization
Numerous libraries and tools can simplify the implementation of authentication and authorization in React applications
1. Firebase Authentication
Firebase Authentication stands as a robust authentication service provided by Google’s Firebase platform. It streamlines the incorporation of authentication into your React application. Let’s delve into a more comprehensive overview of its key features.
User Management:
Firebase Authentication provides a complete user management system. You can handle user registration, sign-in, and password reset flows effortlessly.Social Logins:
It supports various social identity providers like Google, Facebook, Twitter, and more. Users can log in using their existing social media accounts.Email/Password Authentication:
Firebase offers the capability to implement traditional email and password-based authentication systems. This feature plays a pivotal role in ensuring the security of your application’s authentication process by securely hashing and storing user passwords.Custom Authentication:
You can also implement custom authentication flows if your application requires unique user verification methods.Security:
Firebase handles many security aspects, including secure storage of user credentials and protection against common authentication vulnerabilities.Authentication State:
Firebase provides real-time authentication state changes, making it easy to manage different parts of your app based on user authentication status.Integration:
Firebase can be easily integrated into your React application using the Firebase JavaScript SDK.
2. Auth0
Auth0 is an identity and access management platform designed to simplify authentication and authorization. It offers a wide range of features.
Single Sign-On (SSO):
Auth0 supports SSO, allowing users to access multiple applications with a single set of credentials.Social Identity Providers:
Similar to Firebase, Auth0 supports social identity providers, making it easy for users to log in using their social media accounts.Customizable Authentication Flows:
Auth0 allows you to customize the entire authentication process, from sign-up to login, to match your application’s branding and user experience.User Profiles:
You can store additional user profile information in Auth0, which can be useful for personalizing user experiences.Role-Based Access Control (RBAC):
Auth0 includes RBAC features, enabling you to define roles and permissions for users within your application.Multi-Factor Authentication (MFA):
It supports MFA, adding an extra layer of security to user accounts.Integration:
Auth0 can be integrated into your React application using its JavaScript SDK and React SDK.
3. React Context API
Although not specifically designed for authentication, the React Context API is an integral part of React that offers a powerful tool for effectively handling authentication and authorization states within your application.
State Management:
The Context API proves invaluable for establishing a global state that can securely retain authentication and authorization details. This state becomes readily accessible to any component within your application, simplifying the process of managing and sharing critical information across your React application.Context Providers:
Create context providers to wrap your application components, ensuring that authentication-related data is available wherever needed.Avoid Prop Drilling:
The Context API helps eliminate prop drilling, where you pass authentication data through multiple levels of components.Customization:
You have full control over how you structure and manage the authentication context in your React application, allowing for custom solutions tailored to your needs.Integration:
The React Context API is a built-in React feature, so there’s no need to install external libraries. You can start using it right away.
4. React-Query
React-Query is a library that simplifies data-fetching and caching in your React applications. While not directly related to authentication, it can help manage authorization-related data efficiently.
Data Fetching:
React-Query provides a straightforward way to fetch data from APIs and manage that data in your application.Caching:
It offers data caching and synchronization out of the box, reducing the need for unnecessary API calls.Authentication Data:
You can use React-Query to handle data related to user roles, permissions, and other authorization-related information.Optimistic Updates:
React-Query supports optimistic updates, allowing your application to provide a smoother user experience even when data is pending authorization.Integration:
To integrate React-Query into your React application, you can install it as a dependency and configure it to work with your chosen state management solution.
These tools and libraries provide powerful solutions for implementing authentication and authorization in your React application. Depending on your specific requirements and preferences, you can choose the one that best suits your project’s needs. It’s essential to thoroughly research and consider your application’s security and scalability when making your decision.
Also Read: Finding the Perfect Fit: Hiring Dedicated react.js App Developers in the USA
Conclusion
In conclusion, authentication and authorization stand as essential pillars in the construction of secure React applications. Through the diligent implementation of these vital mechanisms, you can fortify user data protection and guarantee that users exclusively access authorized areas within your application.
It’s essential to recognize that security is an ongoing commitment, necessitating a continuous awareness of evolving security best practices and potential vulnerabilities. Crafting a robust authentication and authorization system extends beyond mere recommendation; it becomes an imperative requirement in our contemporary digital landscape, where safeguarding data and user privacy assumes paramount importance.