In the ever-evolving world of web development, staying ahead of the curve is essential. One of the technologies that have gained significant traction is Next.js. If you’re a React developer looking to level up your skills, understanding Next.js fundamentals is a smart move. In this blog, we’ll delve into what Next.js is, why it’s important, and how you can harness its power as a React developer.
What is Next.js?
Next.js is an open-source React framework that simplifies the process of building fast, scalable, and production-ready web applications. Developed by Vercel, Next.js builds upon React’s capabilities and offers additional features that make it a preferred choice for developers, especially in the realm of server-rendered React applications.
Key Features of Next.js
Let’s explore some of the fundamental features that Next.js brings to the table
Server-Side Rendering (SSR)
Next.js excels in server-side rendering, a technique that improves performance by rendering web pages on the server rather than the client’s browser. SSR leads to faster initial page loads and better search engine optimization (SEO). As a React developer, you can harness this feature effortlessly with Next.js.
Automatic Code Splitting
Code splitting is a technique that breaks your code into smaller bundles, loading only what’s necessary for the current page. Next.js handles code splitting automatically, ensuring that your web application is efficient and loads quickly.
Routing Simplified
With Next.js, you get a straightforward routing system. You don’t have to worry about setting up complex route configurations; the framework takes care of it. This simplicity allows you to focus on building your application’s logic.
API Routes
Next.js allows you to create API routes alongside your application. This is incredibly handy for handling server-side logic, making it easier to manage both your frontend and backend within the same project.
Static Site Generation (SSG)
Next.js supports static site generation, a technique where pages are pre-rendered at build time. This is ideal for content-rich websites, blogs, or any application that benefits from delivering static content quickly.
Getting Started with Next.js
Now that you’re familiar with the key features of Next.js, let’s dive into how you can get started:
1. Installation
You can set up a new Next.js project with ease using the `create-next-app` command-line tool. Simply run

2. Pages and Routing
Next.js automatically recognizes the `pages` directory as the entry point for your application. Each file in the `pages` directory corresponds to a route. For example, `pages/index.js` represents the root route, and `pages/about.js` represents an “about” route.
3. Server-Side Rendering
To enable server-side rendering for a page, you can export an `async` function called `getServerSideProps`. This function runs on the server and can fetch data to be passed as props to your component.
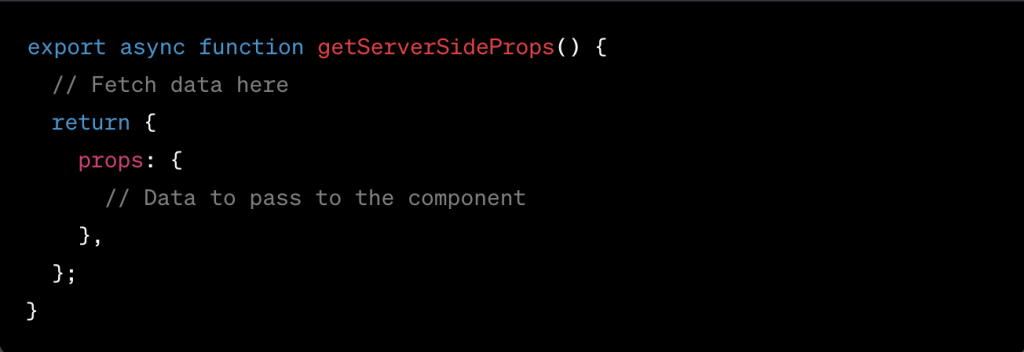
4. Static Site Generation
To enable static site generation for a page, you can export a `getStaticProps` function. This function fetches data at build time and can be used to pre-render pages.
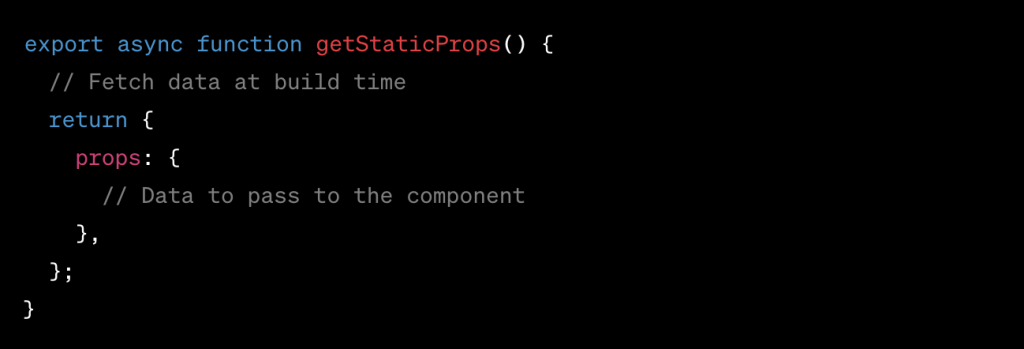
5. Data fetching
Data fetching in Next.js is a versatile and vital feature for React developers. With options like API routes, getStaticProps, and getServerSideProps, Next.js simplifies data retrieval, enabling developers to create fast and SEO-friendly applications while ensuring a smooth user experience.
Also Read: Authentication and Authorization in React Applications
Beyond the Basics
To fully harness the power of Next.js, consider exploring these advanced concepts:
1. Dynamic Routing
Dynamic routing in Next.js allows you to create flexible routes that adapt to varying data. It’s especially useful when you have content that’s fetched from a database or an external API. Here’s what you can explore
Dynamic Route Parameters:
Learn how to define dynamic segments in your route URLs. For example, you can create a route that matches `/products/:id` and fetches product details based on the `id` parameter.
Data Fetching:
Understand how to use the dynamic route parameters to fetch the necessary data for each page. You may need to use functions like `getServerSideProps` or `getStaticProps` to pre-fetch data based on the dynamic route.
Nested Dynamic Routes:
Experiment with nested dynamic routes to create more complex page structures. For instance, you could have routes like `/categories/:category/:product` to display product details within a specific category.
2. Authentication
Implementing authentication in a Next.js application is essential for building secure user experiences. You can explore various approaches to authentication
Third-Party Services:
Integrate authentication providers like Google, Facebook, or GitHub using OAuth or OpenID Connect. Libraries such as `next-auth` can simplify this process.
Custom Authentication:
Build your custom authentication system, which includes user registration, login, and session management. You’ll need to secure routes and manage user sessions.
JWT (JSON Web Tokens):
Learn how to use JWT for token-based authentication. This approach involves issuing tokens upon successful login and verifying them on protected routes.
Authentication UI:
Create user-friendly authentication forms and components. This might involve using UI libraries like `react-query` for data management.
3. Middleware
Middleware functions in Next.js are powerful tools for adding custom logic to your API routes. Here’s how you can explore this concept
Middleware Chains:
Learn how to create middleware chains to execute a series of functions in a specific order before reaching the route handler. This can be useful for tasks like authentication checks, logging, or request parsing.
Route-Specific Middleware:
Implement middleware that’s specific to certain routes. For example, you can apply authentication middleware to protect certain API endpoints while leaving others public.
Error Handling:
Use middleware for global error handling. You can catch and handle errors centrally, making your code cleaner and more maintainable.
4. Internationalization (i18n)
Internationalization support ensures that your Next.js application can cater to a global audience with diverse languages and regions. Here’s what you can explore
Translation Libraries:
Learn about libraries like `react-intl` or `next-translate` that simplify the process of translating your application’s content.
Locale Management:
Understand how to manage locales and language preferences for users. This involves detecting the user’s preferred language and serving content accordingly.
Date and Time Localization:
Implement date and time formatting to display them in the user’s preferred format.
Content Localization:
Explore techniques for translating static content, dynamic data, and even error messages.
5. Deployment
Deploying your Next.js application to a hosting platform ensures that it’s accessible to users worldwide. Consider these deployment options
Vercel:
Vercel is the creator of Next.js and offers seamless deployment. You can connect your GitHub repository to Vercel for automatic deployments whenever you push changes.
Netlify:
Netlify provides straightforward deployment for Next.js apps. It supports serverless functions, making it easy to deploy both frontend and backend code.
Custom Cloud Providers:
If you prefer more control, you can deploy your application on cloud platforms like AWS, Azure, or Google Cloud. This gives you the flexibility to configure your infrastructure as needed.
Continuous Integration (CI/CD):
Explore setting up CI/CD pipelines to automate the deployment process whenever you make changes to your codebase.
Mastering these advanced Next.js concepts will not only enhance your web development skills but also empower you to build feature-rich, performant, and globally accessible applications. As you delve deeper into these topics, you’ll be well-prepared to tackle complex projects and deliver exceptional user experiences.
Related: Next.js App development Tips & Trends to Follow in USA
Best Practices and Pitfalls
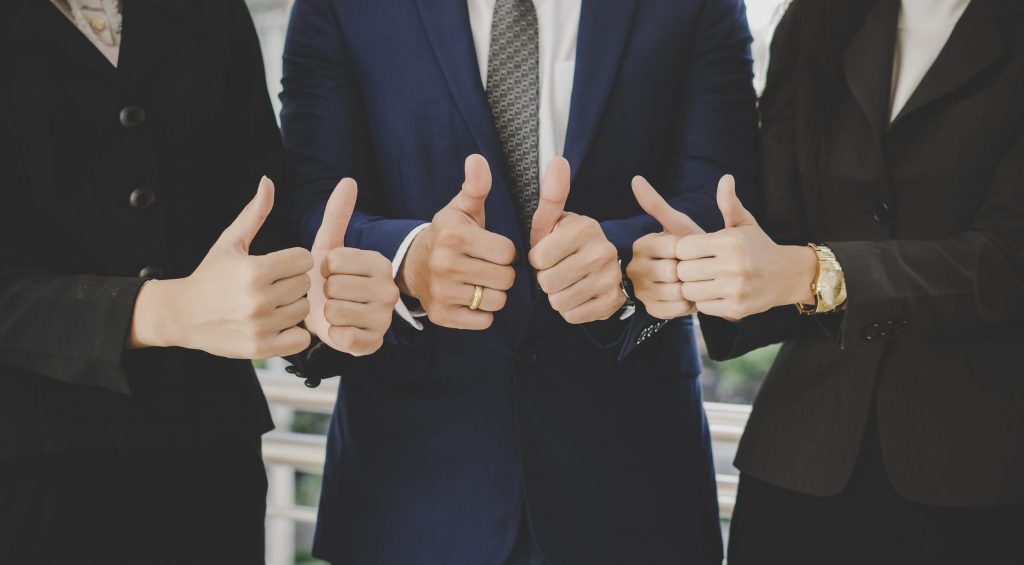
When working with Next.js as a React developer, it’s important to follow best practices to ensure the efficiency and maintainability of your projects. Here are some key guidelines to keep in mind
Best Practices
Code Splitting:
Implement code splitting to divide your JavaScript bundles into smaller chunks. This helps reduce the initial load time of your application, resulting in a better user experience.
Optimize Images:
Use Next.js image optimization to automatically resize and serve images in multiple formats, improving website performance and user experience.
Utilize Prefetching:
Leverage Next.js’s built-in prefetching mechanism to anticipate and load pages that users are likely to visit, enhancing navigation speed.
Cache API Responses:
Implement caching strategies, such as server-side caching or client-side caching with libraries like SWR, to minimize redundant API requests and improve application responsiveness.
Error Handling:
Gracefully handle errors by using custom error pages and meaningful error messages. This ensures that users have a clear understanding of what went wrong.
Responsive Design:
Create responsive layouts to ensure your application looks and functions well on various screen sizes and devices.
Pitfalls to Avoid
Over Fetching Data:
Be cautious not to over fetch data by requesting more information than you need. This can negatively impact performance and increase unnecessary server load.
Excessive Client-Side Rendering:
While client-side rendering is powerful, avoid relying solely on it for content that can be pre-rendered. Balance between SSR and CSR to provide a fast initial load and dynamic client-side interactions.
Ignoring SEO:
Neglecting proper SEO practices, such as setting metadata and optimizing for search engines, can hinder your application’s discoverability on the web.
Not Using `key` Prop:
When rendering lists of items, always provide a unique `key` prop to help React efficiently update the DOM. Failing to do so can lead to unexpected behavior and performance issues.
Heavy Client-Side Logic:
Avoid complex client-side logic that could potentially slow down your application’s interactivity. Consider server-side computations for heavy processing tasks.
Ignoring Security:
Don’t overlook security best practices, such as input validation and authentication checks, to protect your application from potential vulnerabilities.
Conclusion
As a React developer, diving into Next.js fundamentals opens up a world of possibilities. Its robust features, including server-side rendering, automatic code splitting, and simplified routing, can significantly enhance your web development skills. Whether you’re building a personal project or working on a client’s application, Next.js is a powerful tool that can help you deliver faster, more efficient, and SEO-friendly web applications.
So, don’t hesitate to explore the depths of Next.js and elevate your React development game to the next level! The journey may be challenging, but the rewards are boundless. Happy coding!