Testing is an integral part of software development. In the world of web development, React has become one of the most popular JavaScript libraries for building user interfaces. Ensuring that your React applications are free from bugs and issues is crucial for delivering a great user experience. In this blog, we’ll explore various testing techniques and tools to help you test your React applications effectively.
React and Its Advantages
React, developed and maintained by Facebook, has gained immense popularity in the world of web development. It’s a JavaScript library that provides a robust framework for building user interfaces. Here are some of the key advantages of using React
Component-Based Architecture:
React is based on a component-based architecture, where your user interface is broken down into reusable and self-contained components. This approach makes it easier to manage and maintain your codebase. Each component can have its own state and logic, promoting code reusability and modularity.Virtual DOM:
React employs a virtual DOM (Document Object Model) that significantly improves the efficiency of rendering updates. When changes occur in your application, React updates the virtual DOM first, and then it calculates the most efficient way to update the actual DOM. This process minimizes the number of real DOM manipulations, resulting in improved performance and a smoother user experience.Declarative Syntax:
React uses a declarative approach to building user interfaces. This means you describe how your UI should look at any given point in time, and React takes care of updating the actual DOM to match your desired state. This makes your code more predictable and easier to understand, as you don’t have to worry about the step-by-step DOM manipulation that you would perform with an imperative approach.Large and Active Community:
React has a large and active community of developers, which means there is a wealth of resources, libraries, and tools available. You can easily find solutions to common problems, and the library itself is frequently updated with new features and improvements.Cross-Platform Compatibility:
React can be used for both web and mobile app development. React Native, a framework built on top of React, allows you to create native mobile applications for iOS and Android using the same codebase. This cross-platform compatibility saves time and resources when targeting multiple platforms.
Why Testing React Applications is Important
Testing React applications offers several advantages
Bug Prevention:
It helps in identifying and fixing issues early in the development process, reducing the chances of critical bugs making it to production.Code Maintainability:
Well-tested code is more maintainable, as it allows developers to make changes and improvements with confidence.Enhanced Collaboration:
Testing promotes collaboration within development teams, as it makes code more understandable and reliable.Performance Enhancement:
Testing aids in identifying and resolving performance bottlenecks, ensuring that your React app runs smoothly and delivers a faster user experience.Regression Prevention:
Robust testing prevents new code changes from breaking existing features, maintaining your application’s stability over time.
Now, let’s dive into how to test React applications.
Related: Top React Developer Tools for US Developers in 2024
Types of Testing
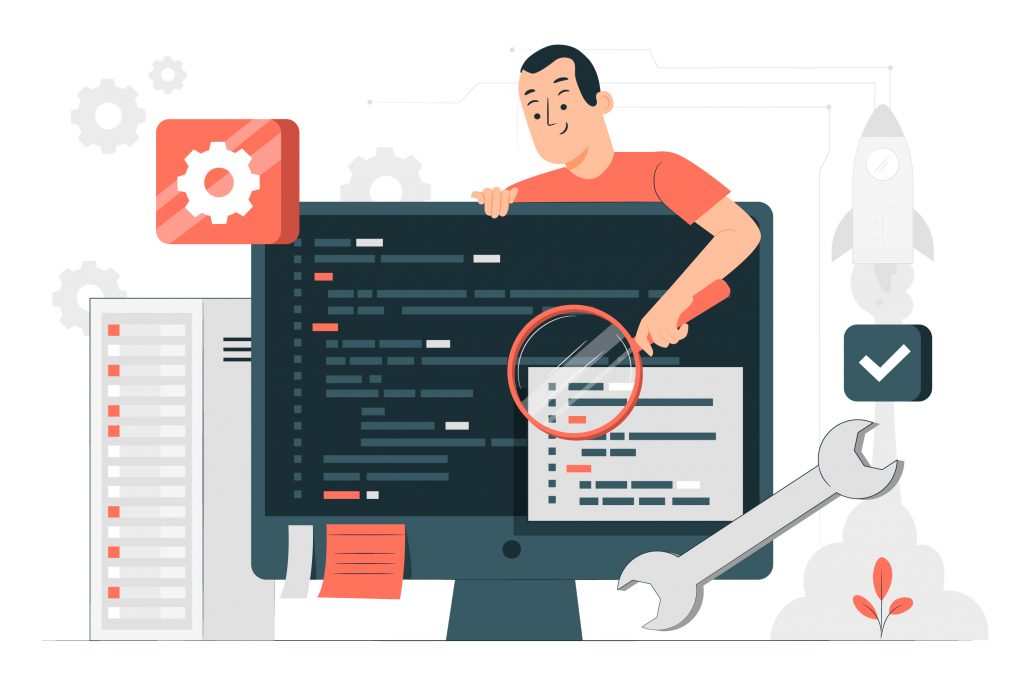
There are several types of testing for React applications. Each serves a specific purpose, and they can be used together for comprehensive coverage.
1. Unit Testing
Unit testing is the practice of testing individual components or functions in isolation to ensure that they perform their intended tasks correctly. In the context of React applications, unit tests focus on testing a single component, function, or module without considering the interactions with other parts of the application. Here’s more information on unit testing in React
Why Unit Testing?
Unit tests are essential for verifying that each component functions correctly. They help identify issues within specific components, such as rendering, state management, and event handling.How It Works:
When writing unit tests for React components, you often use testing libraries like Jest and testing utilities like Enzyme. Mocking is commonly employed to isolate the component from its dependencies. For instance, if a component makes an API call, you’d mock that API call to focus solely on the component’s behavior.What to Test:
In unit testing, you should focus on the component’s input (props and state) and output (rendered output or side effects) and test different scenarios, including edge cases and error conditions.
Unit testing is a fundamental aspect of ensuring the correctness and reliability of your React components, making it easier to catch and fix issues during development.
2. Integration Testing
Integration testing verifies the interactions and collaborations between different parts of your application. It ensures that various components, modules, or services work together cohesively. In React applications, integration testing helps identify issues that might arise when combining multiple components or features. Here’s more about integration testing
Why Integration Testing?
React applications are composed of numerous components, and integration testing ensures that these components interact as expected. It’s especially valuable when you have complex UIs or data flow between components.How It Works:
In integration testing, you test the flow of data and interactions between components, often through user actions or event triggering. You can use tools like React Testing Library or Enzyme to simulate user interactions and verify the expected outcomes.What to Test:
Focus on scenarios where components interact. For example, if you have a form component that interacts with a data-fetching component, you would test the form’s behavior when data is successfully fetched and when there’s an error.
Integration testing ensures that the “glue” between your components works as intended and that the different parts of your application collaborate seamlessly.
3. End-to-End Testing (E2E)
End-to-End (E2E) testing simulates the complete user journey through your application. It ensures that your application behaves correctly from the user’s perspective. E2E testing tools, like Selenium, Cypress, or Playwright, help automate this testing process. Here’s what you need to know about E2E testing
Why E2E Testing?
E2E testing mimics real user interactions, making it an excellent choice for validating that your application’s features work as expected, including UI elements, navigation, and user input.How It Works:
E2E tests typically run in a browser environment, interacting with your application just as a user would. Test scripts simulate actions like clicking buttons, filling forms, and navigating between pages.What to Test:
E2E tests should cover critical user journeys and scenarios, including common and edge cases. For instance, you might test the user registration process, login flow, and a purchase transaction in an e-commerce application.
E2E testing is essential for ensuring that your React application behaves correctly from a user’s perspective and helps catch issues that might not be apparent in unit or integration testing.
4. Snapshot Testing
Snapshot testing is a unique testing approach often used in React with libraries like Jest. It captures and compares a component’s rendered output to a stored “snapshot” of that output. This technique is particularly useful for catching unintended UI changes. Here’s a deeper look into snapshot testing
Why Snapshot Testing?
Snapshot testing helps you detect visual regressions and unintended changes in your application’s user interface. It’s great for ensuring that your UI remains consistent after code changes.How It Works:
When you create a snapshot test for a component, the initial rendering output is saved as a “snapshot.” When the test runs, the current rendering output is compared to the saved snapshot. If there’s a mismatch, the test fails, alerting you to potential UI changes.What to Test:
Snapshot tests are suitable for components with relatively stable UIs, such as presentational components and UI elements. They’re not as helpful for components with dynamic data or complex behavior.
Snapshot testing provides an additional layer of assurance that your UI remains consistent and doesn’t unintentionally change during development.
Testing Libraries and Tools
To effectively test your React applications, you’ll need the right tools. Here are some popular choices
1. Jest
Jest is a popular JavaScript testing framework, often the go-to choice for testing React applications. It was developed by Facebook and is well-suited for unit testing, integration testing, and snapshot testing.
Key Features
Built-in Test Runner:
Jest comes with a built-in test runner that makes it easy to run your test suites and see the results.Assertion Library:
It includes an assertion library for making assertions about your code’s behavior.Snapshot Testing:
Jest is known for its snapshot testing capabilities, which are handy for capturing and verifying the rendered output of components.
Use Cases: Jest is versatile and can be used for various types of testing, from unit tests to integration and snapshot testing. It is often the preferred choice for React developers because it works seamlessly with the library.
2. React Testing Library
React Testing Library is a testing utility specifically designed for React applications. It encourages writing tests that closely simulate how users interact with your components.
Key Features
User-Centric Testing:
This library promotes a user-centric approach to testing, focusing on simulating user interactions and behavior, leading to more realistic and reliable tests.Accessibility Testing:
It provides utilities to test for accessibility (a11y) issues, ensuring your application is usable by individuals with disabilities.DOM Queries:
React Testing Library includes a set of query methods that make it easy to select and interact with elements in the DOM.
Use Cases: React Testing Library is an excellent choice for testing React components, especially when you want to ensure that your components behave as expected from a user’s perspective. It emphasizes testing for accessibility and user experience.
3. Enzyme
Enzyme is a popular testing utility for React, developed by Airbnb. It is particularly useful for rendering and manipulating React components’ output.
Key Features
Shallow Rendering:
Enzyme allows you to perform shallow rendering, which renders only the component under test and not its child components. This can be helpful for isolating the component you want to test.Component Manipulation:
Enzyme provides a set of methods for querying and interacting with components, such as finding elements, simulating events, and inspecting the component’s state and props.Integration with Different Testing Frameworks:
Enzyme can be used with different testing frameworks like Jest, Mocha, or Chai, giving you flexibility in your testing setup.
Use Cases: Enzyme is often chosen when you need fine-grained control over component rendering and interaction. It’s suitable for shallow rendering and for testing how components respond to various input scenarios.
4. Cypress
Cypress is a testing tool specifically designed for end-to-end (E2E) testing of web applications, including React applications. It offers a different approach compared to other tools on this list.
Key Features
E2E Testing:
Cypress excels in E2E testing, allowing you to write tests that simulate user interactions across your application.Real-Time Preview:
Cypress provides a real-time preview of your application as tests run, making it easy to debug and identify issues.Automatic Waiting:
Cypress automatically waits for elements to become interactive, reducing the need for explicit timeouts and ensuring more reliable tests.
Use Cases: Cypress is the tool of choice for E2E testing in React applications. It’s particularly valuable for ensuring that your application’s functionality and user interactions are working correctly.
5. Testing Library (for example, Testing Library for React)
Testing Library is a family of libraries designed for testing user interfaces in a way that mimics how real users interact with your application. For React, you can use libraries like @testing-library/react or @testing-library/react-hooks.
Key Features
User-Centric Approach:
Testing Library encourages writing tests that closely simulate real user interactions, making your tests more realistic and robust.DOM Queries:
It provides a set of query methods to select and interact with elements in the DOM, similar to React Testing Library.Support for Hooks:
Libraries like @testing-library/react-hooks specifically focus on testing custom React hooks, which have become an integral part of many React applications.
Use Cases: Testing Library is particularly useful when you want to ensure that your React components and hooks behave as expected from a user’s perspective. It emphasizes writing tests that closely resemble real-world user interactions, leading to more accurate testing results.
Also Read: 10 React Best Practices to Improve Your Code
Writing Effective Tests
Writing effective tests is as important as choosing the right tools. Here are some tips
Keep Tests isolated:
Effective testing begins with isolating your tests from external dependencies, such as databases or network calls. By using mocking techniques to simulate these external factors, you can ensure that your tests focus solely on the specific functionality or behavior you’re testing. This isolation allows you to identify issues within the code under examination without external factors introducing false positives or negatives.Test Edge Cases:
While it’s important to validate the typical or “happy path” scenarios, it’s equally critical to test edge cases. These edge cases encompass unexpected inputs, error conditions, or exceptional circumstances that your application might encounter. Testing these scenarios helps verify that your code can gracefully handle a wide range of situations, making your application more robust and resilient.Use Descriptive Test Names:
A well-named test can be an invaluable piece of documentation. When your tests have clear and descriptive names, they provide a quick and easily understandable summary of what is being tested. This aids in the maintenance of your test suite and ensures that your fellow developers can quickly grasp the purpose and context of each test case.Continuous Testing:
Continuous testing is a practice that ensures your tests are run automatically whenever changes are pushed to your code repository. Implementing automated testing pipelines in your development workflow helps catch issues early in the development process. By detecting problems as soon as code changes are made, you can address them swiftly, reducing the likelihood of critical bugs reaching production and improving overall code quality.Documentation and Comments:
Adding documentation and comments within your test code is a helpful practice. It clarifies the purpose of the test, the expected outcomes, and any special considerations. This documentation aids not only developers who maintain the tests but also those who might need to understand and work with the test suite in the future. Clear comments provide context and make it easier to troubleshoot and debug failing tests, saving time and effort in the long run.
Conclusion
Testing React applications is crucial for delivering high-quality, bug-free software. It helps you catch issues early in development, reduces maintenance efforts, and boosts collaboration within your development team. With the right testing tools and techniques, you can ensure your React applications are reliable and resilient.
As you continue to develop React applications, remember that testing is an ongoing process. Regularly update and expand your test suite as your application evolves to maintain its quality and reliability. Happy testing!