React has emerged as one of the most popular JavaScript libraries for building user interfaces, and it’s no surprise why. Its component-based architecture, virtual DOM, and robust ecosystem make it an ideal choice for developing efficient and maintainable web applications. However, like any technology, it’s essential to follow best practices to harness React’s full potential and ensure clean, maintainable code. In this blog post, we will explore ten React best practices that can help you improve your code quality, enhance developer productivity, and build more robust applications.
React JS: A Brief Overview
Before diving into best practices, let’s briefly understand what React js. React is an open-source JavaScript library developed by Facebook for building user interfaces. It allows you to create reusable UI components and efficiently manage the state of your application. React’s virtual DOM reconciles changes efficiently, resulting in better performance compared to traditional DOM manipulation.
Benefits of Using React JS
Reusable Components:
React’s component-based architecture promotes reusability. You can create UI components that encapsulate specific functionality, making it easier to maintain and scale your application.
Virtual DOM:
React’s virtual DOM ensures optimal performance by reducing the need for direct DOM manipulation. It updates only the parts of the actual DOM that have changed, resulting in faster rendering.
Strong Ecosystem:
React benefits from a vast ecosystem of libraries, tools, and community support. This ecosystem simplifies various aspects of development, such as routing (React Router) and state management (Redux).
One-Way Data Binding:
React enforces a unidirectional data flow, making it easier to understand how data changes affect your application’s state and UI.
Declarative Syntax:
React uses declarative syntax, which means you describe what you want to render and how your UI should look based on the current application state. This approach makes it easier to understand and reason about your code because it abstracts away the low-level DOM manipulation details, allowing you to focus on the desired UI state.
Performance Optimization:
React’s virtual DOM and efficient diffing algorithm ensure that only the necessary parts of the actual DOM are updated when your application’s state changes. This optimization minimizes the performance bottlenecks associated with frequent re-rendering, resulting in a highly responsive user interface.
Component Reusability:
React encourages the creation of small, reusable components. These components can be composed and reused across different parts of your application or even in other projects. This reusability leads to more maintainable and scalable codebases.
Large Community and Resources:
React has a massive and active community of developers, which means you have access to a wealth of resources, including tutorials, blog posts, videos, and open-source libraries. This community support can significantly accelerate your learning curve and help you find solutions to common challenges.
Server-Side Rendering (SSR) and SEO:
React can be used for server-side rendering (SSR), which improves initial page load times and enhances search engine optimization (SEO). SSR allows your application to render on the server and send fully rendered HTML to the client, which search engines can easily crawl and index.
Compatibility and Integration:
React is designed to be compatible with other JavaScript libraries and frameworks. You can integrate React components seamlessly into existing projects, giving you the flexibility to adopt React incrementally.
Also Read: Finding the Perfect Fit: Hiring Dedicated react.js App Developers in the USA
Challenges Encountered with React JS
While React offers many advantages, it also comes with its set of challenges
Learning Curve:
React’s component-based architecture and its unique way of handling UI development can be initially challenging for developers who are new to the framework. Understanding concepts like components, props, state, and the virtual DOM can take time. However, once developers grasp these fundamentals, they can leverage React’s power to build reusable and maintainable UIs.
Boilerplate Code:
For large-scale React applications, it’s common to find that there’s a need to write boilerplate code. This boilerplate includes setting up components, handling state and props, configuring routing, and managing state with Redux or other state management libraries. While this code is necessary, it can be time-consuming to write and maintain. Developers often use code generators or templates to streamline this process.
Tooling Choices:
The React ecosystem offers a wide range of tools and libraries to choose from, which can be both a blessing and a challenge. Deciding on the right tools, libraries, and dependencies for your project can be daunting. Making informed choices about routing (e.g., React Router), state management (e.g., Redux or MobX), and even styling (e.g., CSS-in-JS or CSS Modules) is crucial. It requires research and an understanding of your project’s specific requirements.
SEO Optimization:
React primarily renders content on the client-side, which can present SEO challenges. Search engines might have difficulty indexing content that relies heavily on client-side rendering. To address this issue, developers often implement server-side rendering (SSR) using tools like Next.js or Gatsby. SSR pre-renders pages on the server and sends fully rendered HTML to improve SEO while still benefiting from React’s component-based approach.
State Management Complexity:
While React provides a built-in state management system through component state, handling complex state logic in larger applications can become challenging. State management libraries like Redux or MobX are often used to centralize and manage application state effectively. However, setting up and maintaining these libraries can introduce additional complexity, especially for developers new to them.
Top React Practices to Improve Code
Now that we’ve covered React’s advantages and challenges, let’s explore the best practices to improve your React code
1. Component Modularity
Break your UI into reusable components: In React, components are the building blocks of your application’s user interface. Aim to create smaller, focused components that encapsulate specific functionality or represent a logical part of your UI. This approach makes your code more maintainable and testable. For example, a complex form can be divided into separate input, validation, and submission components.
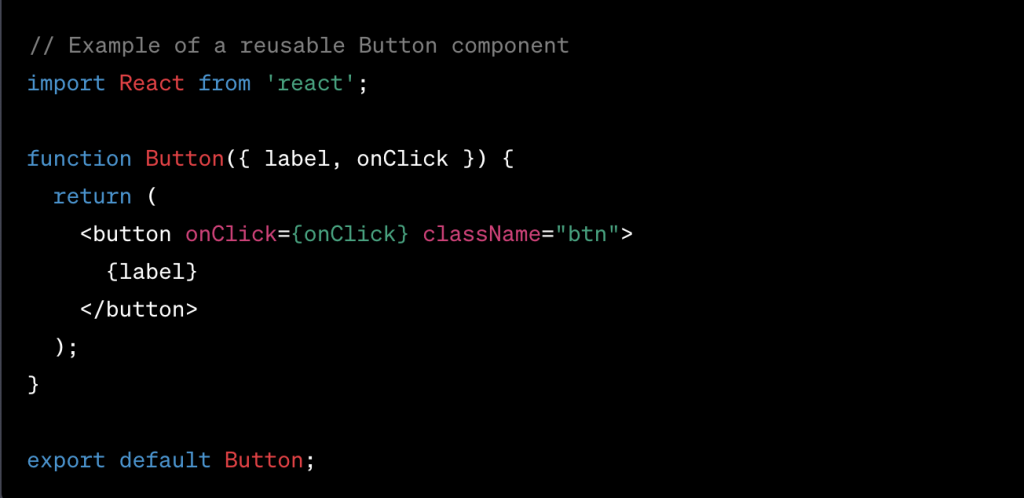
2. Proper State Management
Leverage React’s state management: React provides a built-in way to manage component state. Use local component state when dealing with data specific to that component. For more complex state management scenarios involving data shared between multiple components or global application state, consider using external libraries like Redux or MobX. These libraries help maintain a predictable state flow and simplify data access and updates.
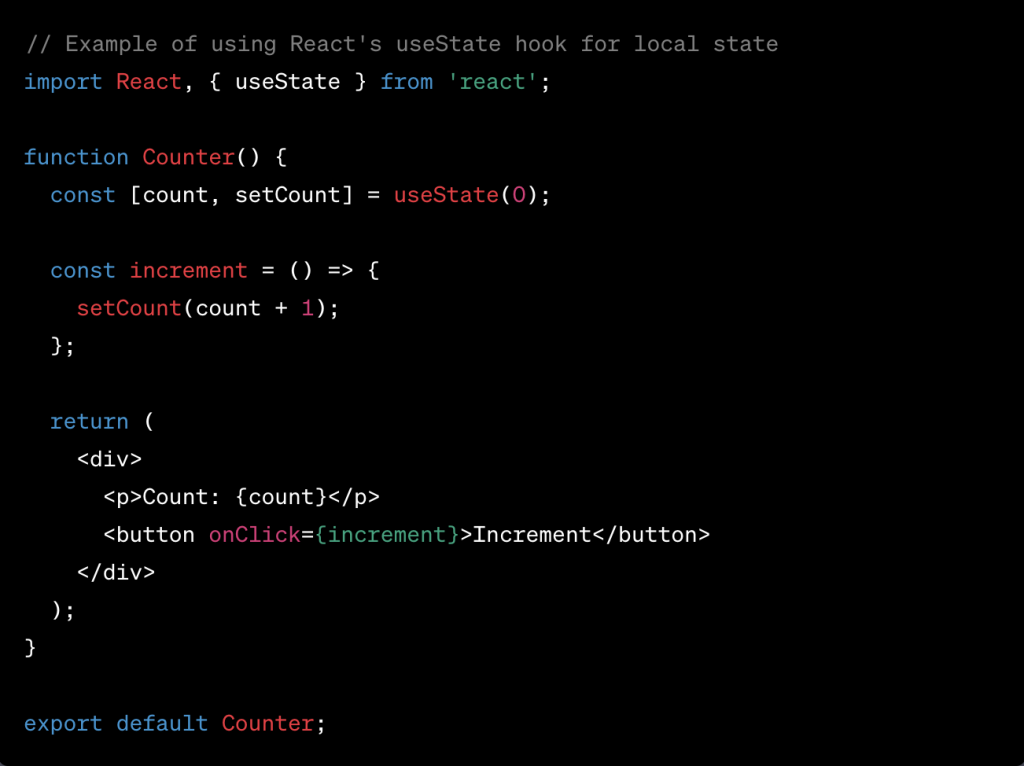
3. Class vs. Functional Components
Prefer functional components with hooks: Functional components, introduced in React with hooks, have become the standard for writing React components. They offer a cleaner and more concise syntax compared to class components. Hooks, such as useState and useEffect, allow you to manage state and perform side effects within functional components, improving code readability and maintainability.
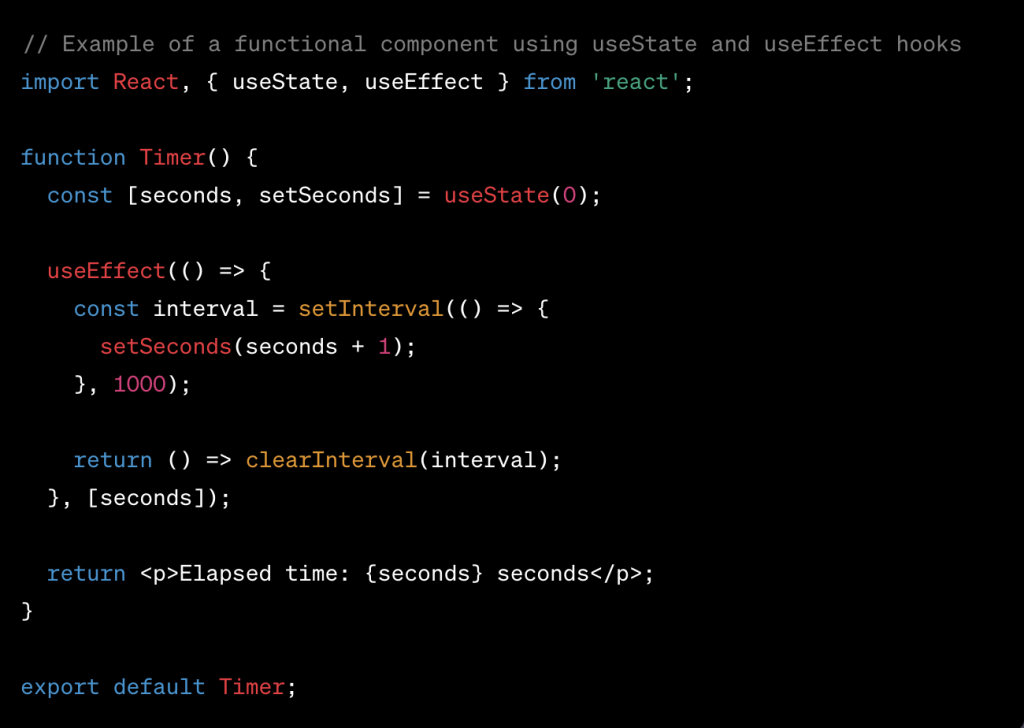
4. PropTypes
Use PropTypes or TypeScript: To ensure strong typing and prevent runtime errors related to component props, use PropTypes or TypeScript. PropTypes are a built-in way to document and validate the expected types of props that a component should receive. TypeScript, on the other hand, provides static type checking for your entire codebase, including React components. Choose the approach that aligns with your project’s needs and preferences.
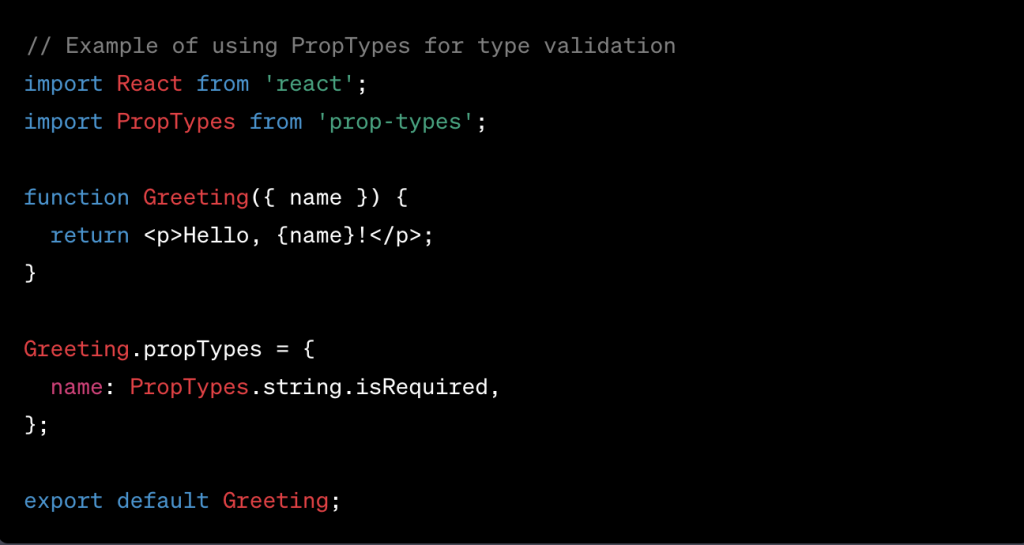
5. Avoid Direct DOM Manipulation
Resist direct DOM manipulation: One of React’s strengths is its virtual DOM, which handles updates efficiently. Avoid manipulating the actual DOM directly using methods like `getElementById` or `querySelector`. Instead, rely on React’s virtual DOM and state management to update and render UI components. Direct DOM manipulation can lead to unexpected behavior and performance issues.
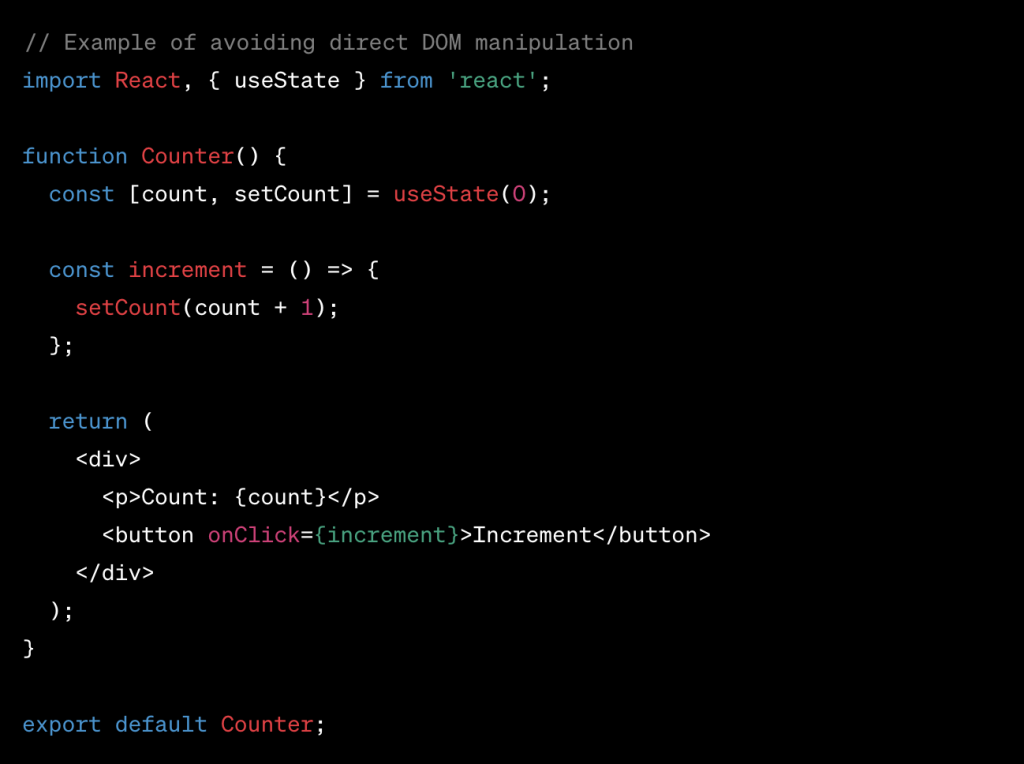
6. Key Props
Provide unique keys for list rendering: When rendering lists of components or elements using the `map` function, always assign a unique `key` prop to each item. React uses these keys to efficiently update and reconcile the virtual DOM, ensuring optimal rendering performance. Keys should be stable and unique for each item in the list.
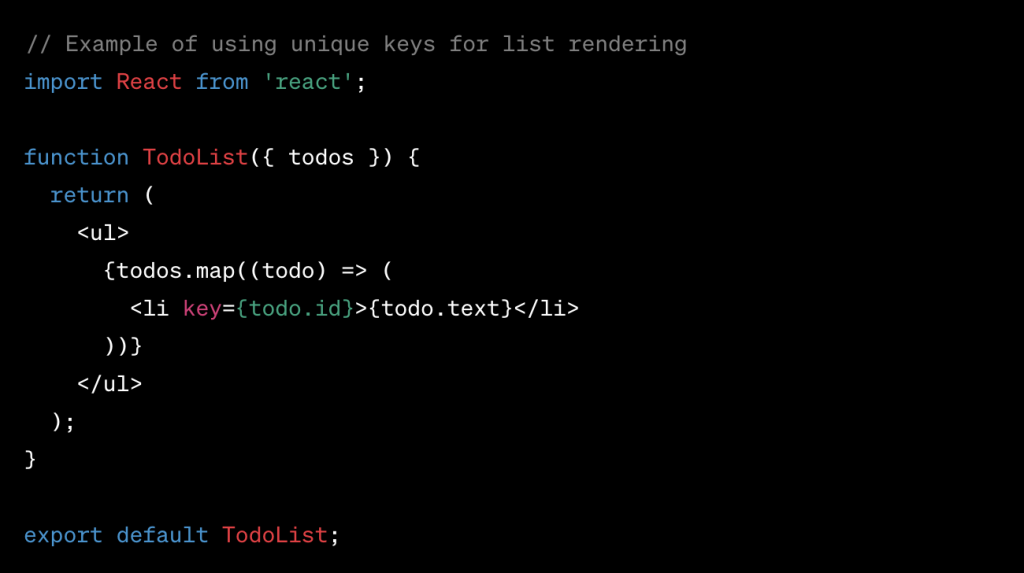
7. Code Splitting
Implement code splitting: To improve page load times and optimize the initial load of your application, use code splitting. This technique involves breaking your JavaScript bundle into smaller chunks that are loaded on-demand as needed. React provides tools like React.lazy and dynamic imports to help with code splitting, allowing you to load only the necessary code for a particular route or feature.
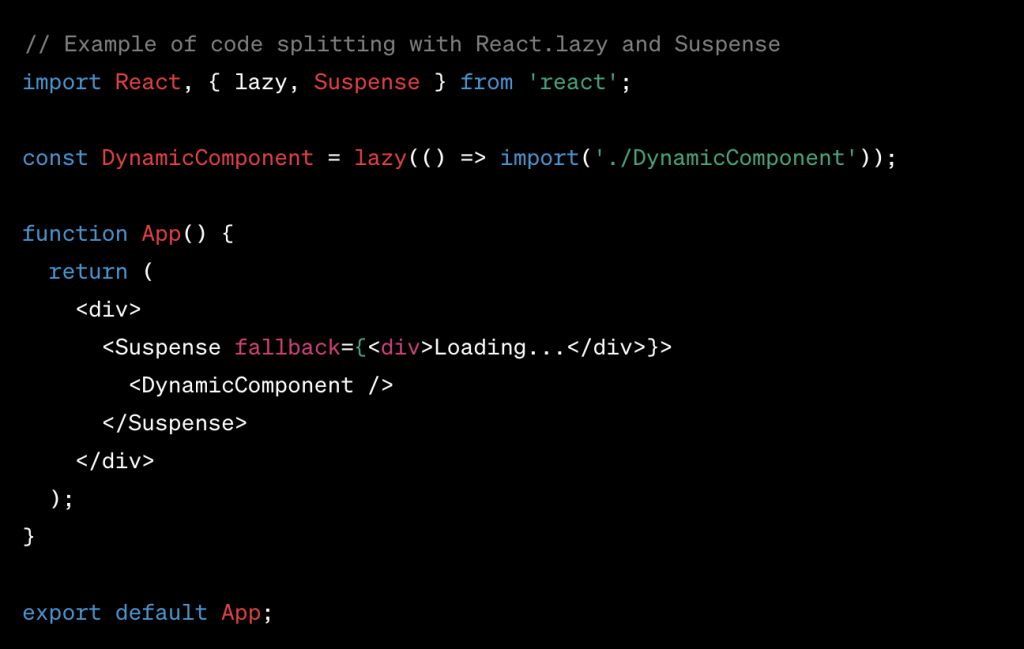
8. CSS-in-JS or CSS Modules
Choose CSS-in-JS or CSS Modules: To encapsulate styles within components and prevent global style conflicts, consider using CSS-in-JS libraries like styled-components or CSS Modules. These approaches allow you to define component-specific styles, making it easier to manage and style your UI components.
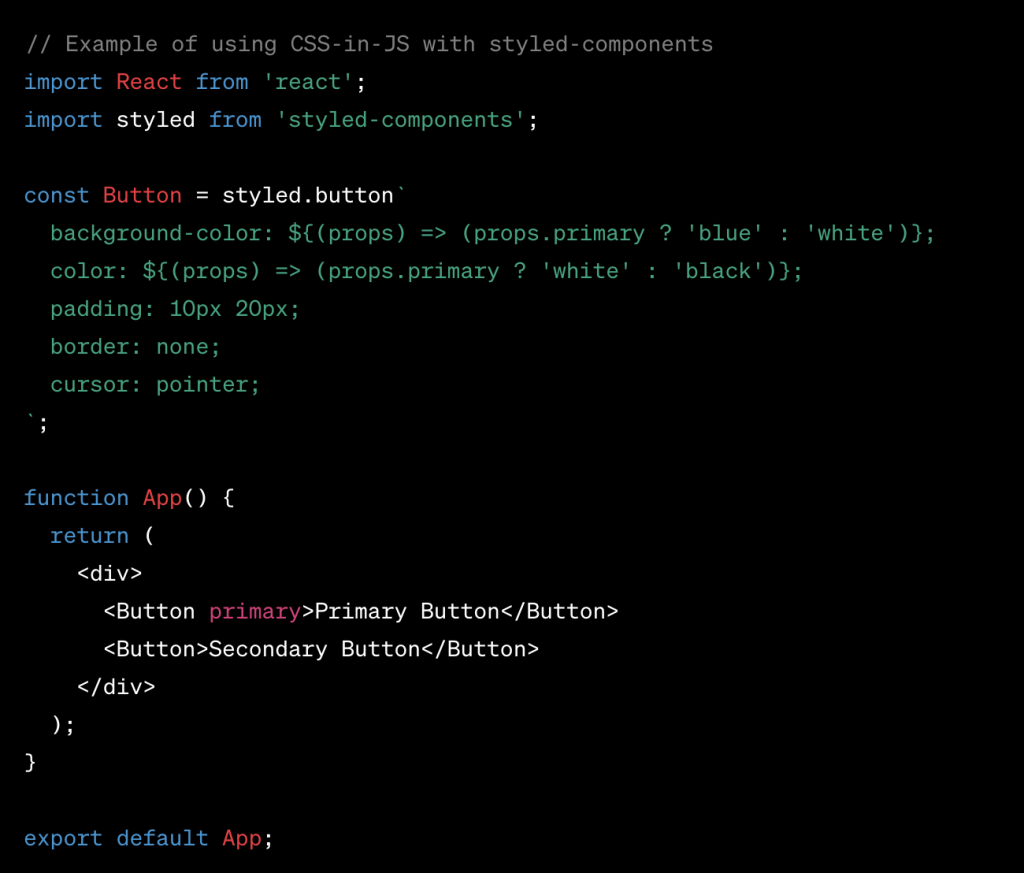
9. Testing
Write unit tests and use testing libraries: Test your React components to ensure their functionality and reliability. Tools like Jest and React Testing Library are popular choices for testing React applications. Write unit tests that cover various scenarios and interactions within your components. Automated testing helps catch issues early in development and maintains code quality.
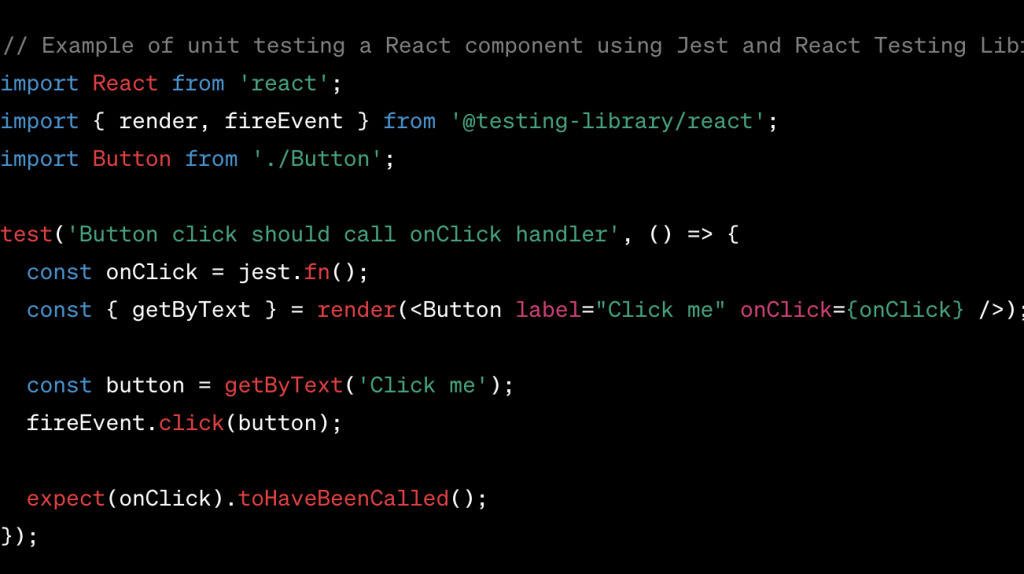
10. Performance Optimization
Optimize performance with profiling: Continuously monitor your application’s performance using tools like React Profiler and browser developer tools. Identify performance bottlenecks, such as unnecessary re-renders or inefficient data fetching, and optimize them. Performance optimization is an ongoing process that ensures your application remains fast and responsive as it evolves.
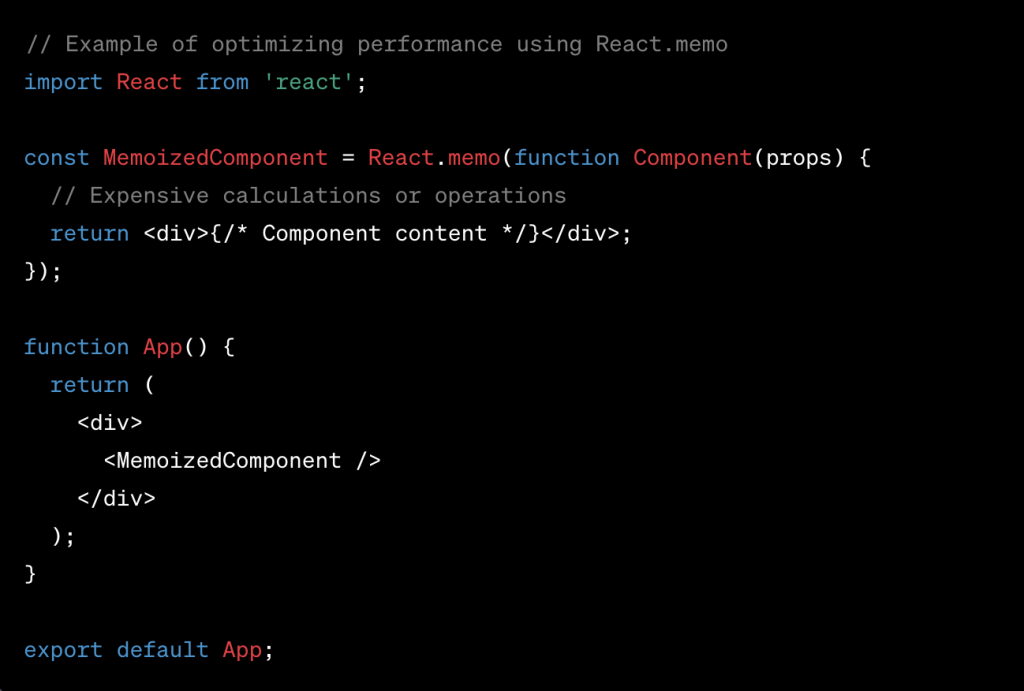
By following these expanded best practices, you can enhance the quality of your React code, improve its maintainability, and create a more efficient and reliable user interface.
Related: The Future of React JS : Top Trends & Predictions
Conclusion
React is a powerful library for building dynamic and interactive user interfaces. By adhering to these ten best practices, you can ensure that your React code is maintainable, efficient, and scalable. Remember that code quality and performance are ongoing concerns, and continuous improvement is key to building successful React applications. Stay up to date with the latest developments in the React ecosystem and be open to exploring new practices that align with your project’s needs. Happy coding!